5th February, 2023
A Service is a special type of Android component used to perform long-running operations in the background. You can play music, handle network transactions and many background operations using this magical Android component. So let’s quick dive into the service.
Android Service
→ Even if user switch to other application, the service will run in background
→ Operating System will only force stop the service when memory is low
→ Service doesn’t provide User Interface (UI)
Types of Android Services
There are three types of Android Services.

Foreground
Services that notify the user about its ongoing operations are called Foreground services. e.g. Downloading a file. If you want to use foreground services, you must have to display a notification so that the user is aware that the service is running.
Background
Background Service doesn’t notify the user about its ongoing operations. User also cannot access them. e.g. data syncing, data storing
Bound
If any component of application is bind with the service, the service is called Bound. You can also binds multiple components. bindService() method is used to bind an application component with a service.
Android Services Lifecycle
The following diagram shows the lifecycle of Android Services. Service have two possible path to complete their lifecycle. Unbounded (Started) and Bounded.
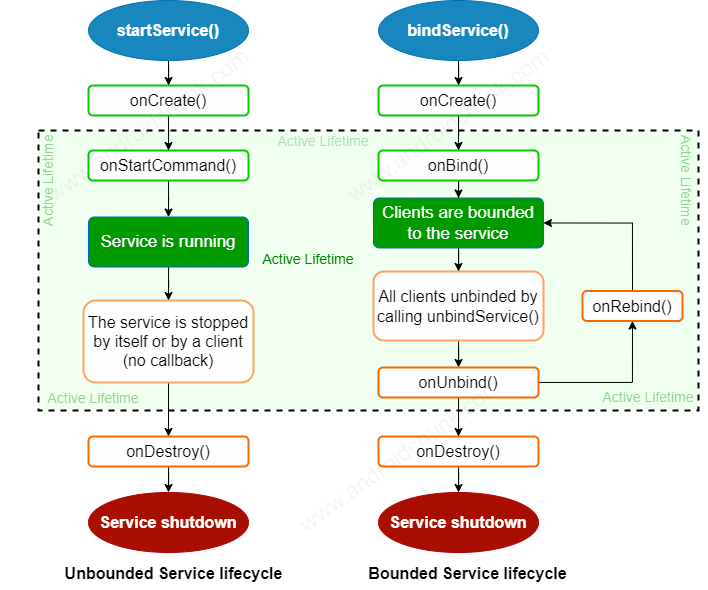
Unbounded Service (Started Service)
A service is started by calling the startService() method using a component. Now the service can run continuously in the background, even if the component that started the service is destroyed.
Unbounded Service (Started Service) can be stopped in two ways:
→ A service can stop itself by calling the stopSelf() method.
→ By calling the stopService() method.
Bounded Service
A component of an application binds itself to a service, it is known as a Bounded service. A component can bind itself to a service by calling the bind() method. Multiple components can bind to a service.
An example of a bounded service is downloading a file from a server. A component starts a service that can be paused, resumed by another component. It is similar to the server-client scenario where the client sends requests and the server responds to the client’s requests.
→ To stop the execution of a Bounded service, all the components must be unbind from the service by calling the unbindService() method.
Android Services Callback Methods
Method | Description |
---|---|
onCreate() | It is required for One-time-Setup. The Android system calls this method when a service is created using onStartCommand() or onBind(). |
onStartCommand() | Android Service calls this method when an activity (a component) starts a service by calling the startService() method. It can be stopped by calling stopSelf() or stopService() methods. |
onBind() | Whenever an application component binds itself to a service using the bindService() method, the onBind() method is invoked. The onBind() method is mandatory to implement in an Android Service. You must provide a User Interface (UI) to communicate with the service by returning an IBinder object, or return null if you don't want binding. |
onUnbind() | The system calls this method when all clients have disconnected from a particular service interface. |
onRebind() | When all clients have disconnected from the interface of the service and new clients have to connected to the service, The system calls this method. |
onDestroy() | When a service is no longer used and being destroyed, The system calls onDestroy() method. Your service must implement onDestroy() method to clean up resources (e.g. register listeners, threads, receivers). |
package com.androidchunk.androidserviceexample import android.app.Service import android.content.Intent import android.os.IBinder class MyService : Service() { // The service is being created override fun onCreate() { super.onCreate() } // Service is ready to start, if you call to startService() override fun onStartCommand(intent: Intent?, flags: Int, startId: Int): Int { return super.onStartCommand(intent, flags, startId) } // A client is binding to the service by calling bindService() override fun onBind(intent: Intent): IBinder? { return null } // when all clients unbound with unbindServices() override fun onUnbind(intent: Intent?): Boolean { return super.onUnbind(intent) } // when a client is bind to the service by calling bindService() // after onUnbind has already been called override fun onRebind(intent: Intent?) { super.onRebind(intent) } // The service is no longer used and is being destroyed override fun onDestroy() { super.onDestroy() } }
package com.androidchunk.androidserviceexample; import android.app.Service; import android.content.Intent; import android.os.IBinder; import androidx.annotation.Nullable; class MyService extends Service { // The service is being created @Override public void onCreate() { super.onCreate(); } // Service is ready to start, if you call to startService() @Override public int onStartCommand(Intent intent, int flags, int startId) { return super.onStartCommand(intent, flags, startId); } // A client is binding to the service by calling bindService() @Nullable @Override public IBinder onBind(Intent intent) { return null; } // when all clients unbound with unbindServices() @Override public boolean onUnbind(Intent intent) { return super.onUnbind(intent); } // when a client is bind to the service by calling bindService() // after onUnbind has already been called @Override public void onRebind(Intent intent) { super.onRebind(intent); } // The service is no longer used and is being destroyed @Override public void onDestroy() { super.onDestroy(); } }
Create Android Service using Android Studio
Open the project tool window and expand go to the directory app ⇒ java and right click on the package (or where you want to create a service class), select New ⇒ Service ⇒ Service.
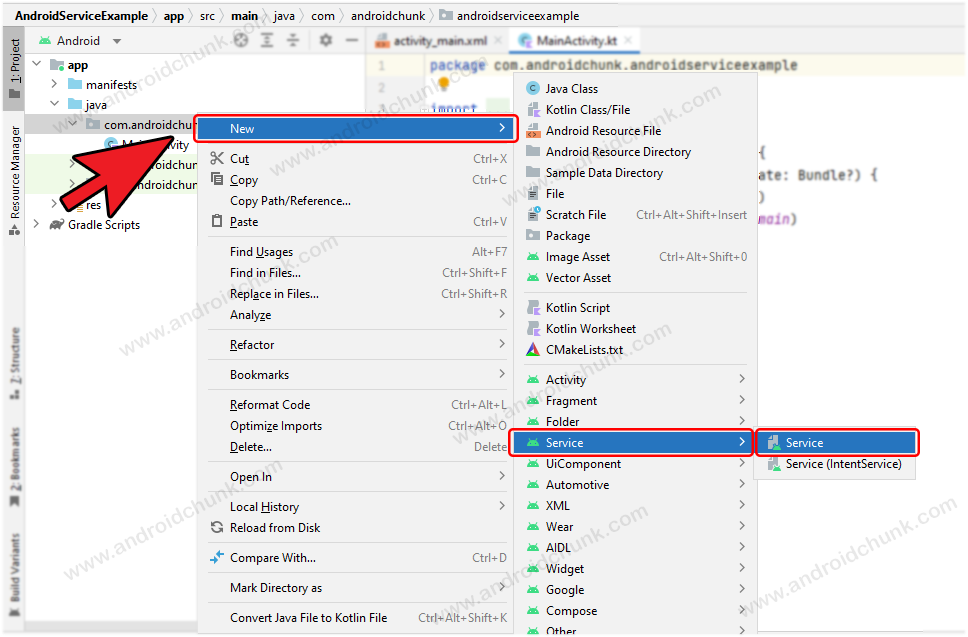
Fill the details of new service class and click the Finish button.
Exported: Other app will use this service if it is true.
Enabled: Indicates that the system can initiate the service or not.

package com.androidchunk.androidserviceexample import android.app.Service import android.content.Intent import android.os.IBinder class MyService : Service() { override fun onBind(intent: Intent): IBinder? { return null } }
package com.androidchunk.androidserviceexample; import android.app.Service; import android.content.Intent; import android.os.IBinder; import androidx.annotation.Nullable; class MyService extends Service { @Nullable @Override public IBinder onBind(Intent intent) { return null; } }
Woohoo! You have successfully created a service.
Register a Service in Manifest File
After creating the service, we have to register it to the androidmanifest.xml file. Luckily Android Studio already accomplished this task for us.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools"> <application> ... ... <service android:name=".MyService" android:enabled="true" android:exported="true" /> <activity/> ... ... </application> </manifest>
package com.androidchunk.androidserviceexample; import android.app.Service; import android.content.Intent; import android.os.IBinder; import androidx.annotation.Nullable; class MyService extends Service { @Nullable @Override public IBinder onBind(Intent intent) { return null; } }
Start a Service
Call startService() method from the Android component (e.g. Activity) to start the Android Service. Have a look at the following code snippet.
var intent = Intent(this, MyService::class.java) startService(intent)
Intent intent = new Intent(this, MyService.class); startService(intent);
Bounded Service vs Unbounded Service vs Intent Service
Unbounded Service (Started Service) | Bounded Service | Intent Service |
Starts by calling the startService() method | Starts by calling the bindService() method | Starts by calling the startService() method |
Stopped or destroyed explicitly by calling stopService() method | Unbind or destroy by calling unbindService() | Implicitly calls stopSelf() to Destroyed |
Basically used to perform long running repetitive task | Basically used to perform long running repetitive task | Basically used to one time task |
Independent of the component from which it starts | Dependent of the component from which it starts | Independent of the component from which it starts |
Happy coding!