14th December, 2022
In this tutorial we will learn one of the most important topics of Android app development, which is Android Activity.
What is Activity?
Activity is referred to as a screen of an Android Application. Activity is one of the building blocks of Android OS. An Android app consists of one or more screens or activities. We can say that Activity is a class pre-written in Kotlin or Java programing.
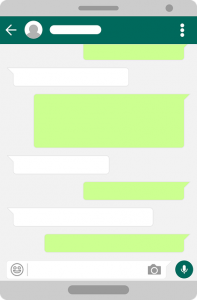
This is an example of a chat screen (activity) of a chatting application. e.g. WhatsApp
This screen will open when the user taps on a chat from the message list or starts a new chat.
You can type a message, view chats, record/send audio messages, and do more using the chat screen
User can also navigate from this screen to another screen(Activity).
In other words, Android Activity provide a frame for users to interact with and perform their tasks.
Android Activity Lifecycle
Every Android Activity goes through a lifecycle or different stages. The various stages of each activity in an Android application are managed by the Android Activity Stack.
There are four major stages in an Activity.
⇒ Running stage
When an activity is shown on the user’s phone screen, it is in the running state.
⇒ Paused state
An Activity is not in focus but still alive for the user. It is in paused state.
⇒ Resumed state
When the Activity goes from paused state to the foreground. This is the resumed state.
⇒ Stopped state
Activity is in the stopped state when it is not in the activity stack and is not visible to the users.
Android Activity Lifecycle Methods
Activity has lifecycle methods like created, started, resumed, paused, stopped or destroyed. Please see the image below that describes the lifecycle of Android Activity.

Method | Description |
---|---|
onCreate() | The onCreate() method is invoked when the activity is first created. It is executed once during the lifetime of the Activity. After onCreate() method, the onStart() method is executed. |
onStart() | Activity is not yet rendered on screen but is about to become visible to the user during the execution of the onStart() method. In this method, you can perform operation related to UI. |
onRestart() | When activity is about to start from the stop state. the onRestart() method is called. This method is to restart an activity that had been active some time back. |
onResume() | When Activity is rendered on the screen, the onResume() method is invoked. Now user can interact with the Activity. Activity is in the Active state. |
onPause() | When Activity losses its focus, the onPause() method is invoked. At this time Activity is going into the background but has not yet been killed. Activity is partially visible to the user. You can commit database transactions and perform light-weight processing. |
onStop() | The onStop() method is invoked when activity is getting destroyed or another existing activity comes back to resume state. Activity is no longer visible to the user. |
onDestroy() | When an activity is destroyed by a user or Android system, the onDestroy() method is invoked. Activity is not in background. |
Take a look at the code of the Android activity lifecycle methods in MainActivity.
package com.example.myapplication1 import android.os.Bundle import androidx.appcompat.app.AppCompatActivity class MainActivity : AppCompatActivity() { override fun onCreate(savedInstanceState: Bundle?) { super.onCreate(savedInstanceState) //Called when the activity is first created } override fun onStart() { super.onStart() //Called after it's creation or by restart method after onStop(). //Activity is not yet rendered on screen //but is about to become visible to the user. //You can perform operation related to UI } override fun onResume() { super.onResume() //Called when Activity is visible to user //Activity is in the active state //user can interact with Activity } override fun onPause() { super.onPause() //Called when Activity losses its focus //Activity is going into the background but has not yet been killed //You can commit database transactions and perform light-weight processing } override fun onStop() { super.onStop() //Called when activity is getting destroyed or //another existing activity comes back to resume state //Activity is no longer visible to the user } override fun onRestart() { super.onRestart() //Called when user comes on screen or resume the activity which was stopped } override fun onDestroy() { super.onDestroy() //Called when an activity is destroyed by a user or Android system //Activity is not in background } }
Declare an Activity In Androidmanifest.xml
To implement in the application we have to register each and every Android activity in Androidmanifest.xml file. The <activity> tag is used to declare an activity.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" > <application android:allowBackup="true" android:dataExtractionRules="@xml/data_extraction_rules" android:fullBackupContent="@xml/backup_rules" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/Theme.MyApplication1" tools:targetApi="31" > <!--Declare an Activity--> <activity android:name=".MainActivity" android:exported="true" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> <meta-data android:name="android.app.lib_name" android:value="" /> </activity> </application> </manifest>
Happy coding!